Akita State Management & Angular
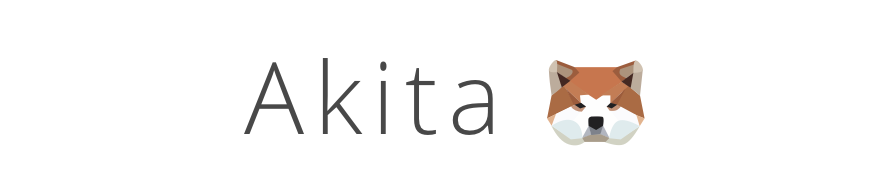
Akita è una libreria di gestione dello stato per Angular, che consente di gestire lo stato dell'applicazione in modo efficiente. Questa libreria segue il pattern Flux, che prevede una gestione unidirezionale dello stato dell'applicazione. In questo articolo, esamineremo in dettaglio come utilizzare Akita su Angular.
1. Installazione di Akita
La prima cosa da fare per utilizzare Akita su Angular è installare la libreria. Questo può essere fatto utilizzando il gestore dei pacchetti npm. Apri il terminale e digita il seguente comando:
npm install @datorama/akita
2. Creazione di uno store
Dopo aver installato Akita, il passo successivo è la creazione di uno store. Lo store è il punto centrale della gestione dello stato dell'applicazione. Per creare uno store, creare una nuova classe TypeScript e importare il Store
e il EntityStore
da Akita.
import { Store, EntityStore } from '@datorama/akita'; export interface Item { id: number; name: string; } export class ItemStore extends EntityStore- { constructor() { super(); } }
La classe ItemStore
estende la classe EntityStore
di Akita e definisce l'interfaccia Item
. Questa interfaccia rappresenta il tipo di oggetto che viene memorizzato nello store. In questo caso, l'oggetto ha due proprietà: id
e name
.
3. Aggiunta di elementi allo store
Dopo aver creato uno store, il passo successivo è aggiungere elementi ad esso. Per aggiungere elementi allo store, utilizzare il metodo add
della classe ItemStore
.
const store = new ItemStore(); store.add([{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }]);
Questo codice crea una nuova istanza di ItemStore
e aggiunge due oggetti di tipo Item
allo store.
4. Recupero di elementi dallo store
Dopo aver aggiunto elementi allo store, il passo successivo è recuperare questi elementi. Per recuperare gli elementi dallo store, utilizzare il metodo selectAll
della classe ItemStore
.
const store = new ItemStore(); store.add([{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }]); const items = store.selectAll(); console.log(items);
Questo codice recupera gli oggetti di tipo Item
dallo store e li stampa sulla console.
5. Modifica degli elementi dello store
Dopo aver recuperato gli elementi dallo store, è possibile modificarli. Per modificare un elemento, utilizzare il metodo update
della classe ItemStore
.
const store = new ItemStore(); store.add([{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }]); store.update(1, { name: 'Item 1 updated' });
Questo codice modifica l'oggetto con l'ID 1 nello store e aggiorna la proprietà name
a "Item 1 updated".
6. Rimozione degli elementi
Dopo aver modificato gli elementi dello store, è possibile rimuoverli. Per rimuovere un elemento, utilizzare il metodo remove
della classe ItemStore
.
const store = new ItemStore(); store.add([{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }]); store.remove(1);
Questo codice rimuove l'oggetto con l'ID 1 dallo store.
7. Gestione degli errori
Akita fornisce anche un modo per gestire gli errori. Per farlo, utilizzare il metodo setError
della classe ItemStore
.
const store = new ItemStore(); store.setError('Error message');
Questo codice imposta un messaggio di errore nello store.
8. Utilizzo di query
Akita fornisce anche una classe Query
che consente di eseguire query sullo store. Per utilizzare la classe Query
, creare una nuova classe TypeScript e importare il Query
da Akita.
import { Query } from '@datorama/akita'; export class ItemQuery extends Query- { constructor(private store: ItemStore) { super(store); } }
La classe ItemQuery
estende la classe Query
di Akita e definisce un costruttore che accetta un'istanza di ItemStore
. Questo costruttore richiama il costruttore della classe base, passando l'istanza di ItemStore
.
Dopo aver creato la classe ItemQuery
, è possibile utilizzare i suoi metodi per eseguire query sullo store. Ad esempio, per recuperare tutti gli elementi con una determinata proprietà, utilizzare il metodo select
della classe ItemQuery
.
const store = new ItemStore(); store.add([{ id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }]); const query = new ItemQuery(store); const items = query.select((item) => item.name === 'Item 1'); console.log(items);
Questo codice crea una nuova istanza di ItemQuery
e utilizza il metodo select
per recuperare gli oggetti di tipo Item
che hanno la proprietà name
uguale a "Item 1".
9. Utilizzo di devtools
Akita fornisce anche un'interfaccia utente per visualizzare lo stato dello store e le azioni che vengono eseguite su di esso. Per utilizzare l'interfaccia utente, installare la libreria @datorama/akita-ngdevtools
utilizzando il gestore dei pacchetti npm
npm install @datorama/akita-ngdevtools --save-dev
Dopo aver installato la libreria, importare il modulo AkitaNgDevtools
nel modulo dell'applicazione e aggiungerlo alla lista dei provider.
import { NgModule } from '@angular/core'; import { AkitaNgDevtools } from '@datorama/akita-ngdevtools'; @NgModule({ imports: [AkitaNgDevtools.forRoot()], }) export class AppModule {}
Conclusione
In questo articolo, abbiamo esaminato in dettaglio come utilizzare Akita su Angular. Abbiamo visto come creare uno store, aggiungere, recuperare, modificare e rimuovere gli elementi dello store e come gestire gli errori. Abbiamo anche visto come utilizzare la classe Query
per eseguire query sullo store e come utilizzare la libreria @datorama/akita-ngdevtools
per visualizzare lo stato dello store e le azioni che vengono eseguite su di esso.
Akita è una libreria molto potente e flessibile per la gestione dello stato delle applicazioni. Offre una soluzione semplice e intuitiva per gestire lo stato delle applicazioni, semplificando il codice e rendendo più facile il debugging.
Sebbene abbia una curva di apprendimento leggermente ripida, Akita fornisce molti vantaggi per gli sviluppatori che cercano una soluzione di gestione dello stato per le loro applicazioni. Con la documentazione dettagliata e la vasta gamma di funzionalità, Akita è un'ottima scelta per lo sviluppo di applicazioni Angular.
English Version
In this article, we will take an in-depth look at how to use Akita with Angular. We will see how to create a store, add, retrieve, modify, and remove items from the store, and how to handle errors. We will also see how to use the Query
class to perform queries on the store and how to use the @datorama/akita-ngdevtools
library to display the state of the store and the actions that are performed on it.
Akita is a powerful and flexible library for managing application state. It provides a simple and intuitive solution for managing application state, simplifying the code and making debugging easier.
Although it has a slightly steep learning curve, Akita provides many advantages for developers looking for a state management solution for their applications. With detailed documentation and a wide range of features, Akita is a great choice for developing Angular applications.
Now, let's dive deeper into how to use Akita with Angular.
To get started, we need to install the @datorama/akita
package:
npm install @datorama/akita
Once installed, we can create a store by extending the EntityStore
class provided by Akita. Here's an example of how to create a simple store for a todo application:
import { EntityState, EntityStore, StoreConfig } from '@datorama/akita'; import { Todo } from './todo.model'; export interface TodoState extends EntityState{ } @StoreConfig({ name: 'todos' }) export class TodoStore extends EntityStore { constructor() { super(); }}
In this example, we define an interface TodoState
that extends the EntityState
provided by Akita. This interface defines the shape of our store state, which in this case consists of a collection of Todo
entities.
Next, we use the @StoreConfig
decorator to define the name of our store. This is used internally by Akita to identify our store and its state.
Finally, we create our TodoStore
class by extending the EntityStore
provided by Akita. We pass in our TodoState
interface and our Todo
model as generic type parameters.
Now that we have created our store, we can use it to manage our application state. We can add, retrieve, modify, and remove items from the store using the methods provided by Akita.
For example, to add a new todo item to our store, we can use the add
method:
const todo = { id: 1, title: 'Buy milk', completed: false }; this.todoStore.add(todo);
To retrieve all the todo items from our store, we can use the selectAll
method:
const todos = this.todoStore.selectAll();
To modify an existing todo item, we can use the update
method:
const updatedTodo = { ...todo, completed: true }; this.todoStore.update(1, updatedTodo);
To remove a todo item from our store, we can use the remove
method:
this.todoStore.remove(1);
In addition to these basic operations, Akita provides many other features for managing application state, such as transactional updates, batching, and lazy loading.
To query our store, we can use the Query
class provided by Akita. Here's an example of how to use Query
to filter the completed todo items:
import { QueryEntity } from '@datorama/akita'; import { Todo } from './todo.model'; import { TodoState, TodoStore } from './todo.store'; @Injectable({ providedIn: 'root' }) export class TodoQuery extends QueryEntity{ constructor(protected store: TodoStore) { super(store); } selectCompleted() { return this.selectAll({ filterBy: todo => todo.completed }); } }
In this example, we define a TodoQuery
class that extends the QueryEntity
provided by Akita. We pass in our TodoState
and Todo
model as generic type parameters, and we inject our TodoStore
instance into the constructor.
We define a custom selectCompleted
method that filters the completed todo items from our store using the filterBy
option provided by Akita.
To use this query, we can inject it into our component or service and call the selectCompleted
method:
constructor(private todoQuery: TodoQuery) {} ngOnInit() { this.completedTodos$ = this.todoQuery.selectCompleted(); }
In this example, we inject our `TodoQuery` instance into our component and use the `selectCompleted` method to get a stream of completed todo items. Finally, let's see how to use the `@datorama/akita-ngdevtools` library to display the state of our store and the actions that are performed on it. To use the `@datorama/akita-ngdevtools` library, we need to install it and import it into our application module:
npm install @datorama/akita-ngdevtools
import { AkitaNgDevtools } from '@datorama/akita-ngdevtools'; @NgModule({ imports: [ environment.production ? [] : AkitaNgDevtools.forRoot(), // other imports ], // other module configurations }) export class AppModule {}
In this example, we import the AkitaNgDevtools
module from the @datorama/akita-ngdevtools
library and add it to our application module. We also conditionally import it based on whether we are in a production environment or not.
Once we have added the AkitaNgDevtools
module, we can open the Akita devtools in our browser by pressing ctrl+shift+k
(or cmd+shift+k
on Mac). This will open a panel that displays the state of our store and the actions that are performed on it.
In conclusion, Akita is a powerful and flexible library for managing application state in Angular. Although it has a slightly steep learning curve, it provides many advantages for developers looking for a state management solution for their applications. With detailed documentation and a wide range of features, Akita is a great choice for developing Angular applications.